Here’s an example of an HTML form that takes input for an RGB color and a JavaScript function that converts the input to its corresponding hexadecimal value:
HTML
RGB to Hex Converter
RGB to Hex Converter
This code defines an HTML form with 3 input fields for red, green, and blue color values (between 0 and 255). Each input field is linked with the convert()
function. I added an additional button “Convert”, so that the user can input all the values and press the convert button to get the result.
The JavaScript convert()
function is defined in the head of the HTML document, it gets the input values, performs bitwise operations and arithmetic to calculate the corresponding hexadecimal value, and assigns the result to the value of the “hex” input field.
When the user inputs a value into one of the color fields and moves away from the field, the JavaScript function is called and the hexadecimal value for the color is calculated and displayed in the “hex” field.
You can run this code by saving it as an HTML file and opening it in a web browser, it will create a simple web form where you can input RGB values and get its corresponding hexadecimal representation.
JAVASCRIPT
Here is a JavaScript function that converts RGB values to their corresponding hexadecimal values:
function convert() {
var r = parseInt(document.getElementById("red").value, 10);
var g = parseInt(document.getElementById("green").value, 10);
var b = parseInt(document.getElementById("blue").value, 10);
var hex = "#" + ((1 << 24) + (r << 16) + (g << 8) + b).toString(16).slice(1);
document.getElementById("hex").value = hex.slice(0, 7);
}
The parseInt() function is used to convert the input values to integers before performing any bitwise operations or arithmetic. This ensures that the values are treated as integers, rather than floating-point numbers, which can produce unexpected results. Also, the hex value is slice to take the first 7 characters, to avoid the numeric overflow.
CSS
/* Add some basic styling to the form */
form {
width: 500px;
margin: 0 auto;
text-align: center;
}
/* Style the labels and input fields */
label {
display: inline-block;
width: 100px;
text-align: right;
margin-right: 10px;
}
input[type="number"] {
width: 50px;
padding: 5px;
margin-bottom: 10px;
}
/* Style the "hex" input field */
input[type="text"] {
width: 120px;
padding: 5px;
margin-bottom: 10px;
background-color: #eee;
}
/* Style the "convert" button */
input[type="button"] {
margin-top: 20px;
padding: 10px 20px;
background-color: #4CAF50;
color: white;
border: none;
cursor: pointer;
}
This code includes an additional <style>
section in the <head>
of the HTML document, where some basic CSS styles are defined to improve the look of the form.
SCREENSHOT
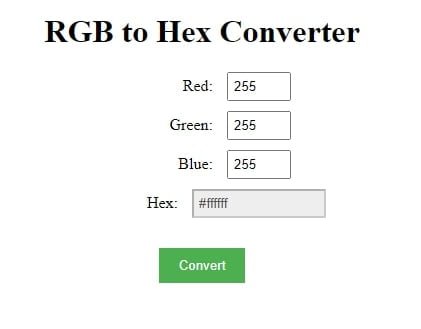